Access thousands of icons as components on-demand universally
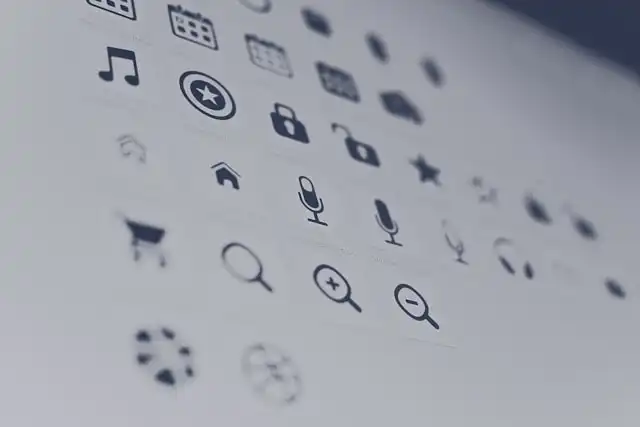
Today I'm showcasing unplugin-icons. An amazing library that basically solved the icon problem for me. Here's a feature list from their README:
Features
- ๐ Universal
- ๐คน Any icon sets - ~150 popular sets with over 200,000 icons, logos, emojis, etc. Powered by Iconify.
- ๐ฆ Major build tools - Vite, Webpack, Rollup, Nuxt, Rspack, etc. Powered by unplugin.
- ๐ Major frameworks - Vanilla, Web Components, React, Vue 3, Vue 2, Solid, Svelte, and more.
- ๐ฑ Any combinations of them!
- โ๏ธ On-demand - Only bundle the icons you really use, while having all the options.
- ๐จ SSR / SSG friendly - Ship the icons with your page, no more FOUC.
- ๐ Stylable - Change size, color, or even add animations as you would with styles and classes.
- ๐ฅ Custom icons - load your custom icons to get universal integrations at ease.
- ๐ฒ Auto Importing - Use icons as components directly in your template.
- ๐ฆพ TypeScript support.
- ๐ Browse Icons
Usage (React)
import IconAccessibility from '~icons/carbon/accessibility';
import IconAccountBox from '~icons/mdi/account-box';
function App() {
return (
<div>
<IconAccessibility />
<IconAccountBox style={{ fontSize: '2em', color: 'red' }} />
</div>
);
}
Installation
Since it's framework agnostic, I'm not gonna be listing all the different ways you can get it to work in your framework of choice. I'll only show how I got it to work in SvelteKit
Install unplugin-icon
pnpm add -D unplugin-icons
Either install the full iconify pack or your icon pack of choice. I'll install the tabler pack:
pnpm add @iconify-json/tabler
In your vite.config.ts
:
import { sveltekit } from '@sveltejs/kit/vite';
import Icons from 'unplugin-icons/vite';
import { defineConfig } from 'vitest/config';
export default defineConfig({
plugins: [
sveltekit(),
Icons({
compiler: 'svelte',
}),
],
});
Finally, in your src/app.d.ts
file:
import 'unplugin-icons/types/svelte';
declare global {
namespace App {}
}
export {};
And that's it! Now you can import the icons across your SvelteKit project with ease:
<script>
import GitHubIcon from 'virtual:icons/tabler/brand-github-filled';
import GoogleIcon from 'virtual:icons/tabler/brand-google-filled';
</script>
<button class="btn btn-error btn-active text-white">
<GoogleIcon width={32} height={32} />
Google
</button>
<button class="btn btn-neutral btn-active">
<GitHubIcon width={32} height={32} />
GitHub
</button>
As you can see, you can also style them because at runtime it's just an svg.
Share